Class CtlTabPage
Introduction
Class for working with tab page controls in a dialog.
EventArgsCallbackT
All EventArgsCallbackT
callbacks include control_src
as a keyword argument.
A callback can be in the format of:
def on_some_event(
src: Any, event: EventArgs, control_src: CtlTabPage, *args, **kwargs
) -> None:
pass
or
def on_some_event(src: Any, event: EventArgs, *args, **kwargs) -> None:
# can get control from kwargs
ctl = cast(CtlTabPage, kwargs['control_src'])
Example
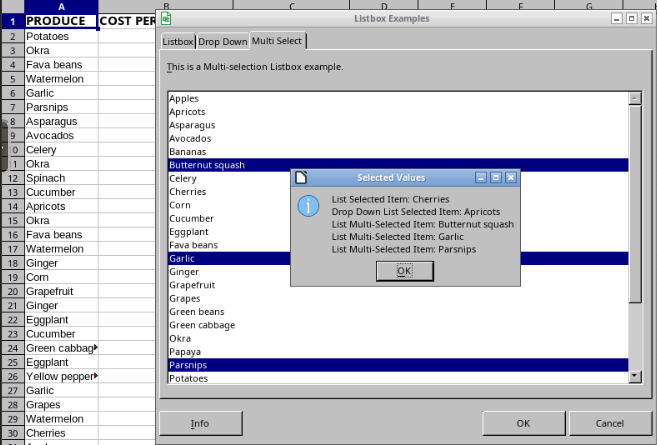
Class
- class ooodev.dialog.dl_control.CtlTabPage(ctl)[source]
Bases:
DialogControlBase
,ContainerEvents
Class for Tab Page Control
- Parameters:
args (Any) –
kwargs (Any) –
- Return type:
Any
- __init__(ctl)[source]
Constructor
- Parameters:
ctl (UnoControlTabPage) – Tab Page Control
- Return type:
None
- add_event_container_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_element_inserted(cb)
Adds a listener for an event.
Event is invoked when a container has inserted an element.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.container.ContainerEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_element_removed(cb)
Adds a listener for an event.
Event is invoked when a container has removed an element.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.container.ContainerEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_element_replaced(cb)
Adds a listener for an event.
Event is invoked when a container has replaced an element.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.container.ContainerEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_focus_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_focus_gained(cb)
Adds a listener for an event.
Event is invoked when a window gains the keyboard focus.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.FocusEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_focus_lost(cb)
Adds a listener for an event.
Event is invoked when a window loses the keyboard focus.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.FocusEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_key_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_key_pressed(cb)
Adds a listener for an event.
Event is invoked when a key has been pressed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.KeyEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_key_released(cb)
Adds a listener for an event.
Event is invoked when a key has been released.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.KeyEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_dragged(cb)
Adds a listener for an event.
Event is invoked when a mouse button is pressed on a window and then dragged.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_entered(cb)
Adds a listener for an event.
Event is invoked when the mouse enters a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_exited(cb)
Adds a listener for an event.
Event is invoked when the mouse exits a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_motion_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_moved(cb)
Adds a listener for an event.
Event Is invoked when the mouse pointer has been moved on a window (with no buttons down).
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_pressed(cb)
Adds a listener for an event.
Event is invoked when a mouse button has been pressed on a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_released(cb)
Adds a listener for an event.
Event is invoked when a mouse button has been released on a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_observers(*args)
Adds observers that gets their
trigger
method called when this classtrigger
method is called.- Parameters:
args (EventObserver) – One or more observers to add.
- Return type:
None
Note
Observers are removed automatically when they are out of scope.
- add_event_paint_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_properties_change(names, cb)
Add properties to listen for changes.
- Parameters:
names (Iterable[str]) – One or more property names to listen for changes.
cb (EventArgsCallbackT) – Callback that is invoked when an event is triggered.
- Raises:
ValueError – If names is empty.
- Return type:
None
Note
The callback
EventArgs.event_data
will contain a tuple ofcom.sun.star.beans.PropertyChangeEvent
objects.Each time this method is called, the previous names are removed and the new names are added.
- add_event_property_change(name, cb)
Adds a listener for an event.
Event is invoked when property is changed.
The callback
EventArgs.event_data
will contain acom.sun.star.beans.PropertyChangeEvent
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_property_change_events_disposing(name, cb)
Adds a listener for an event.
Event is invoked when the property listener is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_vetoable_change(name, cb)
Adds a listener for an event.
Event is invoked when property is changed.
The callback
EventArgs.event_data
will contain acom.sun.star.beans.PropertyChangeEvent
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_vetoable_change_events_disposing(name, cb)
Adds a listener for an event.
Event is invoked when the property listener is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_window_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
Adds a listener for an event.
Event is invoked when the window has been hidden.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_moved(cb)
Adds a listener for an event.
Event is invoked when the window has been moved.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_paint(cb)
Adds a listener for an event.
Event Is invoked when a region of the window became invalid, e.g. when another window has been moved away.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.PaintEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_resized(cb)
Adds a listener for an event.
Event is invoked when the window has been resized.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_shown(cb)
Adds a listener for an event.
Event is invoked when the window has been shown.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- apply_styles(*styles)
Applies styles to control
- Parameters:
*styles (
StyleT
) – Styles to apply- Return type:
None
- fire_event_properties_change(names)
Fires a sequence of PropertyChangeEvents
- Parameters:
names (Iterable[str]) – Sequence of property names to fire event for.
- Return type:
None
- get_control_kind()[source]
Gets the control kind. Returns
DialogControlKind.TAB_PAGE
- Return type:
DialogControlKind
- get_control_named_kind()[source]
Gets the control named kind. Returns
DialogControlNamedKind.TAB_PAGE
- Return type:
DialogControlNamedKind
- get_control_props()
Gets property set for a control model
- Parameters:
control_model (Any) – control model
- Returns:
Property set
- Return type:
XPropertySet | None
- get_property_set()
Gets the property set for this control
- Return type:
XPropertySet
- get_view()
- Return type:
XControl
- remove_event_container_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_element_inserted(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_element_removed(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_element_replaced(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_focus_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_focus_gained(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_focus_lost(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_key_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_key_pressed(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_key_released(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_dragged(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_entered(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_exited(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_motion_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_moved(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_pressed(cb)
Removes a listener for an event.
Event is invoked when a mouse button has been pressed on a window.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_released(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_observer(observer)
Removes an observer
- Parameters:
observer (EventObserver) – One or more observers to add.
- Returns:
True
if observer has been removed; Otherwise,False
.- Return type:
bool
- remove_event_paint_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_properties_listener()
Remove Properties Listener
- Return type:
None
- remove_event_property_change(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_property_change_events_disposing(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_vetoable_change(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_vetoable_change_events_disposing(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_window_events_disposing(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_moved(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_paint(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_resized(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_shown(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- subscribe_event(event_name, callback)
Add an event listener to current instance.
- Parameters:
event_name (str) – Event Name.
callback (EventCallback) – Callback of the event listener.
- Return type:
None
- trigger_event(event_name, event_args)
Trigger an event on current instance.
- Parameters:
event_name (str) – Event Name.
event_args (EventArgsT) – Event Args.
- Return type:
None
- unsubscribe_event(event_name, callback)
Remove an event listener from current instance.
- Parameters:
event_name (str) – Event Name.
callback (EventCallback) – Callback of the event listener.
- Return type:
None
- property event_observer: EventObserver
Gets/Sets The Event Observer for this instance.
- Return type:
- property events_listener_container: ContainerListener
Returns listener
- Return type:
- property events_listener_focus: FocusListener
Returns listener
- Return type:
- property events_listener_key: KeyListener
Returns listener
- Return type:
- property events_listener_mouse: MouseListener
Returns listener
- Return type:
- property events_listener_mouse_motion: MouseMotionListener
Returns listener
- Return type:
- property events_listener_paint: PaintListener
Returns listener
- Return type:
- property events_listener_properties_change_implement: PropertiesChangeListener
Returns listener
- Return type:
- property events_listener_window: WindowListener
Returns listener
- Return type:
- property height: UnitPX
Gets/Sets the height of the control in Pixel units.
When setting can be an integer in
Pixels
Units or aUnitT
.- Returns:
Height of the control.
- Return type:
Note
The Height is in Pixel units; however, the model is in AppFont units. This property will convert the AppFont units to Pixel units. When set using
UnitAppFontHeight
no conversion is done.
- property help_text: str
Gets/Sets the tip text
- Return type:
str
- property horizontal_scrollbar: bool
Gets or sets if a horizontal scrollbar should be added to the dialog.
Returns
False
if LibreOffice version is less than7.1
since
LibreOffice
7.1
- Return type:
bool
- property model: com.sun.star.awt.tab.UnoControlTabPageModel
Uno Control Model
- Return type:
UnoControlTabPageModel
- property name: str
Gets/Sets the name of the control.
- Return type:
str
- property scroll_height: int
Gets or sets the total height of the scrollable dialog content
Returns
-1
if LibreOffice version is less than7.1
since
LibreOffice
7.1
- Return type:
int
- property scroll_left: int
Gets or sets the horizontal position of the scrolled dialog content
Returns
-1
if LibreOffice version is less than7.1
since
LibreOffice
7.1
- Return type:
int
- property scroll_top: int
Gets or sets the vertical position of the scrolled dialog content
Returns
-1
if LibreOffice version is less than7.1
since
LibreOffice
7.1
- Return type:
int
- property scroll_width: int
Gets or sets the total width of the scrollable dialog content
Returns
-1
if LibreOffice version is less than7.1
since
LibreOffice
7.1
- Return type:
int
- property step: int
Gets/Sets the step
- Return type:
int
- property tab_index: int
Gets/Sets the tab index
- Return type:
int
- property tag: str
Gets/Sets the tag
- Return type:
str
- property tip_text: str
Gets/Sets the tip text
- Return type:
str
- property title: str
Gets or sets the title of the tab page
- Return type:
str
- property vertical_scrollbar: bool
Gets or sets if a vertical scrollbar should be added to the dialog.
Returns
False
if LibreOffice version is less than7.1
since
LibreOffice
7.1
- Return type:
bool
- property view: com.sun.star.awt.tab.UnoControlTabPage
Uno Control
- Return type:
UnoControlTabPage
- property visible: bool
Gets/Sets the visible state for the control
- Return type:
bool
- property x: UnitPX
Gets/Sets the
X
position for the control in pixel units.When setting can be an integer in
Pixels
Units or aUnitT
.- Returns:
X position of the control.
- Return type:
Note
The
X
is in Pixel units; however, the model is in AppFont units. This property will convert the AppFont units to Pixel units. When set usingUnitAppFontX
no conversion is done.
- property y: UnitPX
Gets/Sets the
Y
position for the control in pixel units.When setting can be an integer in
Pixels
Units or aUnitT
.- Returns:
Y position of the control.
- Return type:
Note
The
Y
is in Pixel units; however, the model is in AppFont units. This property will convert the AppFont units to Pixel units. When set usingUnitAppFontY
no conversion is done.