Write Modify Page Header Transparency
The ooodev.format.writer.modify.page.header.transparency.Transparency
and ooodev.format.writer.modify.page.header.transparency.Gradient
classes are used to modify the Area style values seen in Fig. 1146 of a Page style.
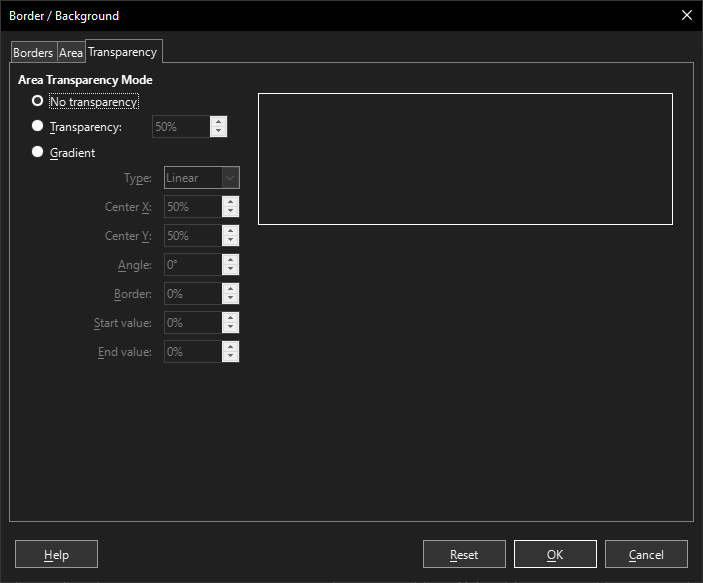
Fig. 1146 Writer dialog Header Transparency default
Default Page Style Dialog
Setup
General function used to run these examples.
from ooodev.format.writer.modify.page.header import Header, WriterStylePageKind
from ooodev.format.writer.modify.page.header.transparency import (
Transparency,
Gradient,
IntensityRange,
GradientStyle,
)
from ooodev.format.writer.modify.page.header.area import Color as HeaderAreaColor
from ooodev.format import Styler
from ooodev.utils.color import StandardColor
from ooodev.office.write import Write
from ooodev.gui import GUI
from ooodev.loader.lo import Lo
def main() -> int:
with Lo.Loader(Lo.ConnectPipe()):
doc = Write.create_doc()
GUI.set_visible(doc=doc)
Lo.delay(300)
GUI.zoom(GUI.ZoomEnum.ENTIRE_PAGE)
header_style = Header(
on=True,
shared_first=True,
shared=True,
height=10.0,
spacing=3.0,
spacing_dyn=True,
margin_left=1.5,
margin_right=2.0,
style_name=WriterStylePageKind.STANDARD,
)
page_header_style_kind = WriterStylePageKind.STANDARD
color_style = HeaderAreaColor(color=StandardColor.RED, style_name=page_header_style_kind)
transparency_style = Transparency(value=85, style_name=page_header_style_kind)
Styler.apply(doc, header_style, color_style, transparency_style)
style_obj = Transparency.from_style(doc=doc, style_name=WriterStylePageKind.STANDARD)
assert style_obj.prop_style_name == str(WriterStylePageKind.STANDARD)
Lo.delay(1_000)
Lo.close_doc(doc)
return 0
if __name__ == "__main__":
SystemExit(main())
Transparency
The Transparency
class is used to modify the transparency of a page header style.
The result are seen in Fig. 1147 and Fig. 1148.
Setting Transparency
In this example we will apply a transparency to the page header style background color.
The transparency needs to be applied after the page header style color as the transparency is applied to the color.
This means the order Styler.apply(doc, header_style, color_style, transparency_style)
is important.
The transparency is set to 85% in this example.
# ... other code
page_header_style_kind = WriterStylePageKind.STANDARD
color_style = HeaderAreaColor(color=StandardColor.RED, style_name=page_header_style_kind)
transparency_style = Transparency(value=85, style_name=page_header_style_kind)
Styler.apply(doc, header_style, color_style, transparency_style)
Style results.
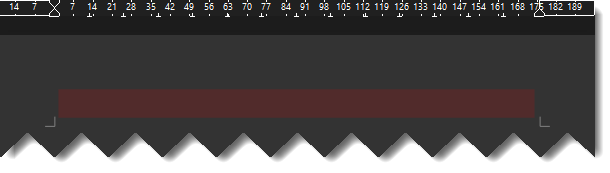
Fig. 1147 Writer Page Header
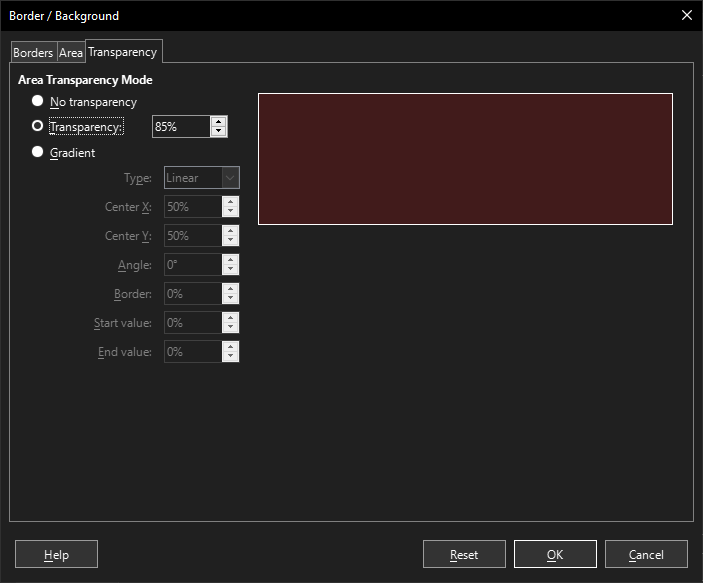
Fig. 1148 Writer dialog Page Header Transparency style changed
Getting transparency from a style
# ... other code
style_obj = Transparency.from_style(doc=doc, style_name=WriterStylePageKind.STANDARD)
assert style_obj.prop_style_name == str(WriterStylePageKind.STANDARD)
Transparency Gradient
Setting Transparency Gradient
The Gradient
class is used to modify the area gradient of a page header style.
The result are seen in Fig. 1149 and Fig. 1150.
In this example we will apply a transparency to the page header style background color.
The transparency needs to be applied after the page header style color as the transparency is applied to the color.
This means the order Styler.apply(doc, header_style, color_style, header_gradient_style)
is important.
# ... other code
page_header_style_kind = WriterStylePageKind.STANDARD
color_style = HeaderAreaColor(color=StandardColor.GREEN_DARK1, style_name=page_header_style_kind)
header_gradient_style = Gradient(
style=GradientStyle.LINEAR,
angle=45,
border=22,
grad_intensity=IntensityRange(0, 100),
style_name=page_header_style_kind,
)
Styler.apply(doc, header_style, color_style, header_gradient_style)
Style results.
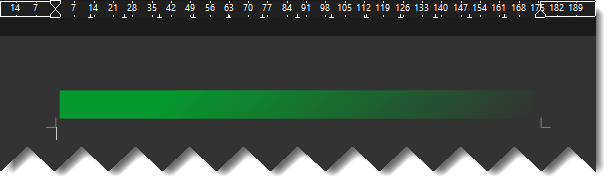
Fig. 1149 Writer Page Header
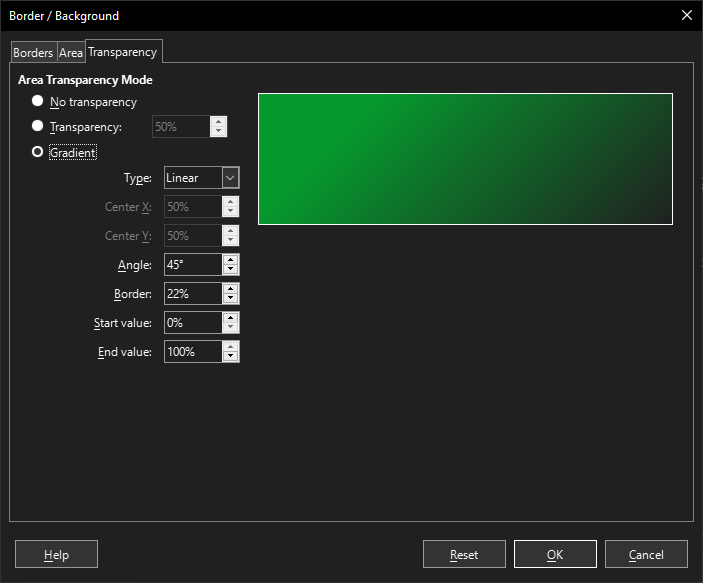
Fig. 1150 Writer dialog Page Header Transparency style changed
Getting gradient from a style
# ... other code
style_obj = Gradient.from_style(doc=doc, style_name=WriterStylePageKind.STANDARD)
assert style_obj.prop_style_name == str(WriterStylePageKind.STANDARD)