Class CtlTextEdit
Introduction
Class for working with text edit controls in a dialog.
EventArgsCallbackT
All EventArgsCallbackT
callbacks include control_src
as a keyword argument.
A callback can be in the format of:
def on_some_event(
src: Any, event: EventArgs, control_src: CtlTextEdit, *args, **kwargs
) -> None:
pass
or
def on_some_event(src: Any, event: EventArgs, *args, **kwargs) -> None:
# can get control from kwargs
ctl = cast(CtlTextEdit, kwargs['control_src'])
Example
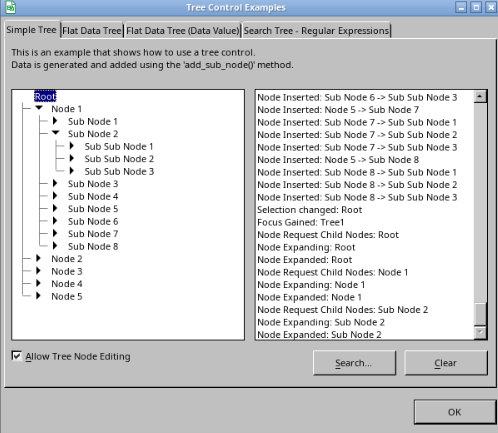
For an example see Tab and Tree Dialog Example
Class
- class ooodev.dialog.dl_control.CtlTextEdit(ctl)[source]
Bases:
DialogControlBase
,UnoControlEditModelPartial
,TextEvents
Class for Text Edit Control
- __init__(ctl)[source]
Constructor
- Parameters:
ctl (UnoControlEdit) – Button Control
- Return type:
None
- add_event_focus_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_focus_gained(cb)
Adds a listener for an event.
Event is invoked when a window gains the keyboard focus.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.FocusEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_focus_lost(cb)
Adds a listener for an event.
Event is invoked when a window loses the keyboard focus.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.FocusEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_key_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_key_pressed(cb)
Adds a listener for an event.
Event is invoked when a key has been pressed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.KeyEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_key_released(cb)
Adds a listener for an event.
Event is invoked when a key has been released.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.KeyEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_listener(listener)
Adds an event listener to the component.
- Parameters:
listener (XEventListener) – The event listener to be added.
- Return type:
None
- add_event_mouse_dragged(cb)
Adds a listener for an event.
Event is invoked when a mouse button is pressed on a window and then dragged.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_entered(cb)
Adds a listener for an event.
Event is invoked when the mouse enters a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_exited(cb)
Adds a listener for an event.
Event is invoked when the mouse exits a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_motion_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_moved(cb)
Adds a listener for an event.
Event Is invoked when the mouse pointer has been moved on a window (with no buttons down).
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_pressed(cb)
Adds a listener for an event.
Event is invoked when a mouse button has been pressed on a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_mouse_released(cb)
Adds a listener for an event.
Event is invoked when a mouse button has been released on a window.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.MouseEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_observers(*args)
Adds observers that gets their
trigger
method called when this classtrigger
method is called.- Parameters:
args (EventObserver) – One or more observers to add.
- Return type:
None
Note
Observers are removed automatically when they are out of scope.
- add_event_paint_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_properties_change(names, cb)
Add properties to listen for changes.
- Parameters:
names (Iterable[str]) – One or more property names to listen for changes.
cb (EventArgsCallbackT) – Callback that is invoked when an event is triggered.
- Raises:
ValueError – If names is empty.
- Return type:
None
Note
The callback
EventArgs.event_data
will contain a tuple ofcom.sun.star.beans.PropertyChangeEvent
objects.Each time this method is called, the previous names are removed and the new names are added.
- add_event_property_change(name, cb)
Adds a listener for an event.
Event is invoked when property is changed.
The callback
EventArgs.event_data
will contain acom.sun.star.beans.PropertyChangeEvent
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_property_change_events_disposing(name, cb)
Adds a listener for an event.
Event is invoked when the property listener is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_text_changed(cb)
Adds a listener for an event.
Event is invoked when the text has changed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.TextEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_text_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_vetoable_change(name, cb)
Adds a listener for an event.
Event is invoked when property is changed.
The callback
EventArgs.event_data
will contain acom.sun.star.beans.PropertyChangeEvent
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_vetoable_change_events_disposing(name, cb)
Adds a listener for an event.
Event is invoked when the property listener is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Parameters:
name (str) – Property Name
cb (EventArgsCallbackT) – Callback
- Return type:
None
- add_event_window_events_disposing(cb)
Adds a listener for an event.
Event is invoked when the broadcaster is about to be disposed.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.lang.EventObject
struct.- Return type:
None
- Parameters:
cb (Any) –
Adds a listener for an event.
Event is invoked when the window has been hidden.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_moved(cb)
Adds a listener for an event.
Event is invoked when the window has been moved.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_paint(cb)
Adds a listener for an event.
Event Is invoked when a region of the window became invalid, e.g. when another window has been moved away.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.PaintEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_resized(cb)
Adds a listener for an event.
Event is invoked when the window has been resized.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_event_window_shown(cb)
Adds a listener for an event.
Event is invoked when the window has been shown.
The callback
EventArgs.event_data
will contain a UNOcom.sun.star.awt.WindowEvent
struct.- Return type:
None
- Parameters:
cb (Any) –
- add_property_change_listener(name, listener)
Adds a listener for property changes.
- Parameters:
name (str) – The name of the property.
listener (Any) – The listener to be added.
- Return type:
None
- add_vetoable_change_listener(name, listener)
Adds a listener for vetoable changes.
- Parameters:
name (str) – The name of the property.
listener (Any) – The listener to be added.
- Return type:
None
- apply_styles(*styles)
Applies styles to control
- Parameters:
*styles (
StyleT
) – Styles to apply- Return type:
None
- static create(win, **kwargs)[source]
Creates a new instance of the control.
Keyword arguments are optional. Extra Keyword args are passed to the control as property values.
- Parameters:
win (XWindowPeer) – Parent Window
kwargs (Any) –
- Keyword Arguments:
- Returns:
New instance of the control.
- Return type:
Note
The UnoControlDialogElement interface is not included when creating the control with a window peer.
- create_clone()
Creates a clone of the object.
- Returns:
The clone.
- Return type:
XCloneable
- dispose()
Disposes the component.
- Return type:
None
- fire_event_properties_change(names)
Fires a sequence of PropertyChangeEvents
- Parameters:
names (Iterable[str]) – Sequence of property names to fire event for.
- Return type:
None
- get_control_kind()[source]
Gets the control kind. Returns
DialogControlKind.EDIT
- Return type:
DialogControlKind
- get_control_named_kind()[source]
Gets the control named kind. Returns
DialogControlNamedKind.EDIT
- Return type:
DialogControlNamedKind
- get_control_props()
Gets property set for a control model
- Parameters:
control_model (Any) – control model
- Returns:
Property set
- Return type:
XPropertySet | None
- get_property_set()
Gets the property set for this control
- Return type:
XPropertySet
- get_property_set_info()
Returns the property set info.
- Returns:
The property set info.
- Return type:
XPropertySetInfo
- get_property_value(name)
Returns the value of a property.
- Parameters:
name (str) – The name of the property.
- Returns:
The value of the property.
- Return type:
Any
- get_view()
- Return type:
XControl
- remove_event_focus_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_focus_gained(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_focus_lost(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_key_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_key_pressed(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_key_released(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_listener(listener)
Removes an event listener from the component.
- Parameters:
listener (XEventListener) – The event listener to be removed.
- Return type:
None
- remove_event_mouse_dragged(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_entered(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_exited(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_motion_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_moved(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_pressed(cb)
Removes a listener for an event.
Event is invoked when a mouse button has been pressed on a window.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_mouse_released(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_observer(observer)
Removes an observer
- Parameters:
observer (EventObserver) – One or more observers to add.
- Returns:
True
if observer has been removed; Otherwise,False
.- Return type:
bool
- remove_event_paint_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_properties_listener()
Remove Properties Listener
- Return type:
None
- remove_event_property_change(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_property_change_events_disposing(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_text_changed(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_text_events_disposing(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_vetoable_change(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_vetoable_change_events_disposing(name)
Removes a listener for an event
- Parameters:
name (str) – Property Name
- Return type:
None
- remove_event_window_events_disposing(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_moved(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_paint(cb)
Removes a listener for an event
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_resized(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_event_window_shown(cb)
Removes a listener for an event.
- Return type:
None
- Parameters:
cb (Any) –
- remove_property_change_listener(name, listener)
Removes a listener for property changes.
- Parameters:
name (str) – The name of the property.
listener (Any) – The listener to be removed.
- Return type:
None
- remove_vetoable_change_listener(name, listener)
Removes a listener for vetoable changes.
- Parameters:
name (str) – The name of the property.
listener (Any) – The listener to be removed.
- Return type:
None
- set_font_descriptor(font_descriptor)
Sets the font descriptor of the control.
- Parameters:
font_descriptor (FontDescriptor, FontDescriptorStructComp) – UNO Struct - Font descriptor to set.
- Return type:
None
Note
The
font_descriptor
property can also be used to set the font descriptor.Hint
FontDescriptor
can be imported fromooo.dyn.awt.font_descriptor
.
- Return type:
None
- Parameters:
font_descriptor (FontDescriptor | FontDescriptorStructComp) –
- set_property_value(name, value)
Sets the value of a property.
- Parameters:
name (str) – The name of the property.
value (Any) – The value of the property.
- Return type:
None
- subscribe_event(event_name, callback)
Add an event listener to current instance.
- Parameters:
event_name (str) – Event Name.
callback (EventCallback) – Callback of the event listener.
- Return type:
None
- trigger_event(event_name, event_args)
Trigger an event on current instance.
- Parameters:
event_name (str) – Event Name.
event_args (EventArgsT) – Event Args.
- Return type:
None
- unsubscribe_event(event_name, callback)
Remove an event listener from current instance.
- Parameters:
event_name (str) – Event Name.
callback (EventCallback) – Callback of the event listener.
- Return type:
None
- write_line(line='')[source]
Add a new line to a multi-line text control
- Parameters:
line (str, optional) – Specifies a line to insert at the end of the text box a newline character will be inserted before the line, if relevant.
- Returns:
True if successful, False otherwise
- Return type:
bool
- property align: AlignKind
Get/Sets the horizontal alignment of the text in the control.
Hint
AlignKind
can be imported fromooodev.utils.kind.align_kind
.
- Return type:
AlignKind
- property auto_h_scroll: bool | None
Gets/Sets - If set to
True
an horizontal scrollbar will be added automatically when needed.optional
- Return type:
bool | None
- property auto_v_scroll: bool | None
Gets/Sets - If set to
True
a vertical scrollbar will be added automatically when needed.optional
- Return type:
bool | None
- property background_color: Color
Gets/Set the background color of the control.
- Returns:
Color
- Return type:
- property border: BorderKind
Gets/Sets the border style of the control.
Note
Value can be set with
BorderKind
orint
.Hint
BorderKind
can be imported fromooodev.utils.kind.border_kind
.
- Return type:
- property border_color: Color
Gets/Sets the color of the border, if present
Not every border style (see Border) may support coloring. For instance, usually a border with 3D effect will ignore the border_color setting.
- Returns:
Color
- Return type:
- property echo_char: str
Gets/Sets the echo character as a string
- Return type:
str
- property enabled: bool
Gets/Sets whether the control is enabled or disabled.
- Return type:
bool
- property event_observer: EventObserver
Gets/Sets The Event Observer for this instance.
- Return type:
- property events_listener_focus: FocusListener
Returns listener
- Return type:
- property events_listener_key: KeyListener
Returns listener
- Return type:
- property events_listener_mouse: MouseListener
Returns listener
- Return type:
- property events_listener_mouse_motion: MouseMotionListener
Returns listener
- Return type:
- property events_listener_paint: PaintListener
Returns listener
- Return type:
- property events_listener_properties_change_implement: PropertiesChangeListener
Returns listener
- Return type:
- property events_listener_text: TextListener
Returns listener
- Return type:
- property events_listener_window: WindowListener
Returns listener
- Return type:
- property font_descriptor: FontDescriptorStructComp
Gets/Sets the Font Descriptor.
Setting value can be done with a
FontDescriptor
orFontDescriptorStructComp
object.- Returns:
Font Descriptor
- Return type:
Hint
FontDescriptor
can be imported fromooo.dyn.awt.font_descriptor
.
- property font_emphasis_mark: FontEmphasisEnum
Gets/Sets the
FontEmphasis
value of the text in the control.Note
Value can be set with
FontEmphasisEnum
orint
.Hint
FontEmphasisEnum
can be imported fromooo.dyn.text.font_emphasis
.
- Return type:
FontEmphasisEnum
- property font_relief: FontReliefEnum
Gets/Sets
FontRelief
value of the text in the control.Note
Value can be set with
FontReliefEnum
orint
.Hint
FontReliefEnum
can be imported fromooo.dyn.text.font_relief
.
- Return type:
FontReliefEnum
- property h_scroll: bool
Gets/Sets if the content of the control can be scrolled in the horizontal direction.
- Return type:
bool
- property hard_line_breaks: bool
Gets/Sets if hard line breaks will be returned in the
XTextComponent.getText()
method.- Return type:
bool
- property help_text: str
Gets/Sets the tip text
- Return type:
str
- property help_url: str
Gets/Sets the help URL of the control.
- Return type:
str
- property hide_inactive_selection: bool | None
Gets/Sets whether the selection in the control should be hidden when the control is not active (focused).
optional
- Return type:
bool | None
- property line_end_format: LineEndFormatEnum | None
specifies which line end type should be used for multi line text
Controls working with this model care for this setting when the user enters text. Every line break entered into the control will be treated according to this setting, so that the Text property always contains only line ends in the format specified.
Possible values are all constants from the LineEndFormat group.
Note that this setting is usually not relevant when you set new text via the API. No matter which line end format is used in this new text then, usual control implementations should recognize all line end formats and display them properly.
optional
Note
Value can be set with
LineEndFormatEnum
orint
.Hint
LineEndFormatEnum
can be imported fromooo.dyn.awt.line_end_format
.
- Return type:
LineEndFormatEnum | None
- property max_text_len: int
Gets/Sets the maximum character count.
There’s no limitation, if set to 0.
- Return type:
int
- property model: com.sun.star.awt.UnoControlEditModel
Uno Control Model
- Return type:
UnoControlEditModel
- property model_ex: ModelTextEdit
Gets the extended Model for the control.
This is a wrapped instance for the model property. It add some additional properties and methods to the model.
- Return type:
ModelTextEdit
- property multi_line: bool
Gets/Sets that the text may be displayed on more than one line.
- Return type:
bool
- property paint_transparent: bool | None
Gets/Sets whether the control paints it background or not.
optional
- Return type:
bool | None
- property printable: bool
Gets/Sets that the control will be printed with the document.
- Return type:
bool
- property read_only: bool
Gets/Sets if the content of the control cannot be modified by the user.
- Return type:
bool
- property tabstop: bool
Gets/Sets if the control can be reached with the TAB key.
- Return type:
bool
- property text: str
Gets/Sets the text displayed in the control.
- Return type:
str
- property text_color: Color
Gets/Sets the text color of the control.
- Return type:
NewType()
(Color
,int
)
- property text_line_color: Color
Gets/Sets the text line color (RGB) of the control.
- Return type:
NewType()
(Color
,int
)
- property tip_text: str
Gets/Sets the tip text
- Return type:
str
- property v_scroll: bool
Gets/Sets if the content of the control can be scrolled in the vertical direction.
- Return type:
bool
- property vertical_align: VerticalAlignment | None
Gets/Sets the vertical alignment of the text in the control.
optional
Hint
VerticalAlignment
can be imported fromooo.dyn.style.vertical_alignment
- Return type:
VerticalAlignment | None
- property view: com.sun.star.awt.UnoControlEdit
Uno Control
- Return type:
UnoControlEdit
- property view_ex: ViewTextEdit
Gets the extended View for the control.
This is a wrapped instance for the view property. It add some additional properties and methods to the view.
- Return type:
- property visible: bool
Gets/Sets the visible state for the control
- Return type:
bool
- property writing_mode: int | None
Denotes the writing mode used in the control, as specified in the
com.sun.star.text.WritingMode2
constants group.Only LR_TB (
0
) and RL_TB (1
) are supported at the moment.optional
- Return type:
int | None