Write Style Para Class
Overview
The ooodev.format.writer.style.Para
class is used to set the paragraph style.
Chapter 6. Text Styles has more information on paragraph styles.
Setup
General function used to run these examples.
import uno
from com.sun.star.text import XTextDocument
from ooodev.format.writer.direct.char.font import Font
from ooodev.format.writer.direct.para.area import Color as ParaBgColor
from ooodev.format.writer.direct.para.indent_space import Spacing, LineSpacing, ModeKind
from ooodev.format.writer.style import Para as StylePara
from ooodev.office.write import Write
from ooodev.units import UnitMM
from ooodev.utils.color import StandardColor
from ooodev.gui import GUI
from ooodev.loader.lo import Lo
def main() -> int:
p_txt = (
"To Sherlock Holmes she is always THE woman. I have seldom heard"
" him mention her under any other name. In his eyes she eclipses"
" and predominates the whole of her sex. It was not that he felt"
" any emotion akin to love for Irene Adler. All emotions, and that"
" one particularly, were abhorrent to his cold, precise but"
" admirably balanced mind. He was, I take it, the most perfect"
" reasoning and observing machine that the world has seen, but as a"
" lover he would have placed himself in a false position. He never"
" spoke of the softer passions, save with a gibe and a sneer. They"
" were admirable things for the observer--excellent for drawing the"
" veil from men's motives and actions. But for the trained reasoner"
" to admit such intrusions into his own delicate and finely"
" adjusted temperament was to introduce a distracting factor which"
" might throw a doubt upon all his mental results. Grit in a"
" sensitive instrument, or a crack in one of his own high-power"
" lenses, would not be more disturbing than a strong emotion in a"
" nature such as his. And yet there was but one woman to him, and"
" that woman was the late Irene Adler, of dubious and questionable memory."
)
with Lo.Loader(Lo.ConnectPipe()):
doc = Write.create_doc()
GUI.set_visible(doc=doc)
Lo.delay(300)
GUI.zoom(GUI.ZoomEnum.ENTIRE_PAGE)
new_style_name = "CoolParagraph"
if not create_para_style(doc, new_style_name):
raise RuntimeError(f"Could not create new paragraph style: {new_style_name}")
# get the XTextRange of the document
xtext_range = doc.getText().getStart()
# Load the paragraph style and apply it to the text range.
para_style = StylePara(new_style_name)
para_style.apply(xtext_range)
cursor = Write.get_cursor(doc)
Write.append_para(cursor=cursor, text=p_txt)
Lo.delay(1_000)
Lo.close_doc(doc)
return 0
def create_para_style(doc: XTextDocument, style_name: str) -> bool:
try:
# font style for the paragraph.
font = Font(
name="Liberation Serif", size=12.0, color=StandardColor.PURPLE_DARK2, b=True
)
# spacing below paragraph
spc = Spacing(below=UnitMM(4))
# paragraph line spacing
ln_spc = LineSpacing(mode=ModeKind.FIXED, value=UnitMM(6))
# paragraph background color
bg_color = ParaBgColor(color=StandardColor.GREEN_LIGHT2)
_ = Write.create_style_para(
text_doc=doc,
style_name=style_name,
styles=[font, spc, ln_spc, bg_color]
)
return True
except Exception as e:
print("Could not set paragraph style")
print(f" {e}")
return False
if __name__ == "__main__":
SystemExit(main())
Examples
Style a paragraph with a custom style
Create a new paragraph style, assign it to a paragraph and set the paragraph style.
See 6.4 Applying Styles to Paragraphs (and Characters) for more information.
# ... other code
new_style_name = "CoolParagraph"
if not create_para_style(doc, new_style_name):
raise RuntimeError(f"Could not create new paragraph style: {new_style_name}")
# get the XTextRange of the document
xtext_range = doc.getText().getStart()
# Load the paragraph style and apply it to the text range.
para_style = StylePara(new_style_name)
para_style.apply(xtext_range)
# ... other code
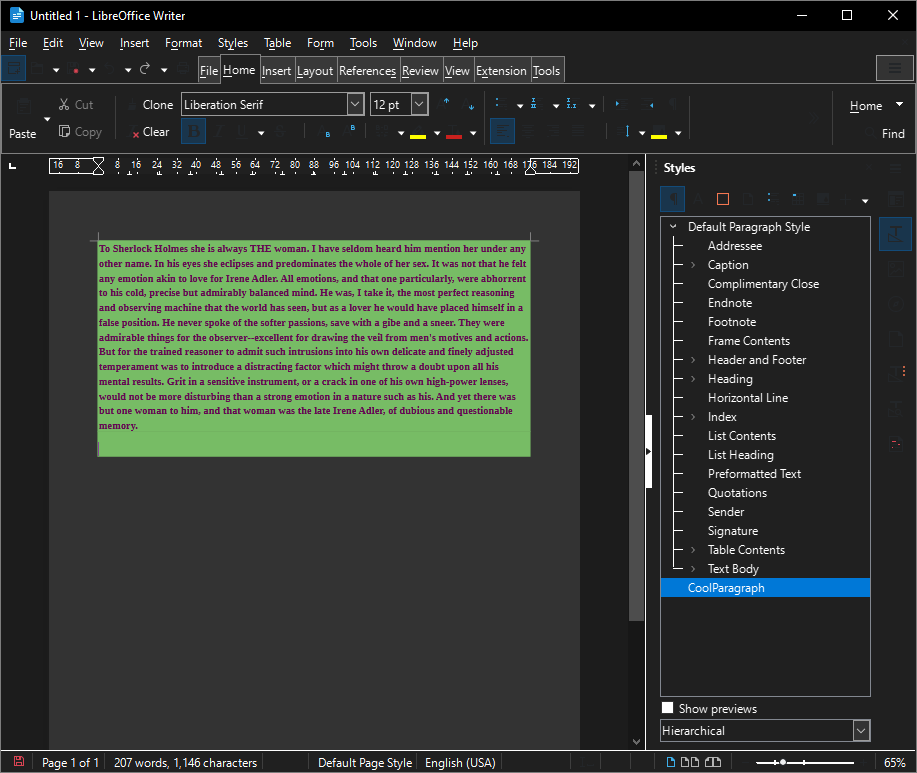
Fig. 1178 Newly styled paragraph.
Resetting Paragraph Style to Default
By accessing the static property Para.default
the default paragraph style can be accessed
which is a default style that is set to default values.
This can be used to reset the paragraph style to the default style.
from ooodev.format.writer.style import Para as StylePara
# ... other code
StylePara.default.apply(cursor)
Write.append_para(cursor=cursor, text="Back to default style.")
# ... other code
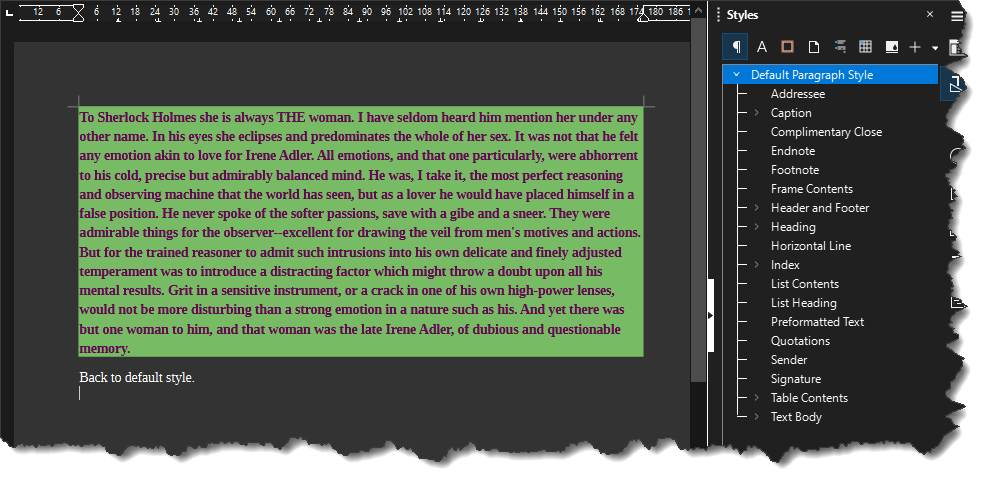
Fig. 1179 Paragraph style reset to default.
Getting style from paragraph
By accessing the static property Para.default
the default paragraph style can be accessed
which is a default style that is set to default values.
This can be used to reset the paragraph style to the default style.
from ooodev.format.writer.style import Para as StylePara
# ... other code
para_cursor = Write.get_paragraph_cursor(cursor)
para_cursor.gotoPreviousParagraph(False)
para_cursor.gotoEndOfParagraph(True)
para_style = StylePara.from_obj(para_cursor)
# assert name of paragraph style is CoolParagraph
assert para_style.prop_name == new_style_name
# ... other code