Calc Direct Cell Protection
Overview
The style_protection()
method is called to set the cell protection properties.
The style_protection()
class gives you the same options as Calc’s Cell Protection Dialog tab,
but without the dialog. as seen in Fig. 342.
Warning
Note that cell protection is not the same as sheet protection and cell protection is only enabled with sheet protection is enabled. See LibreOffice Help - Cell Protection for more information.
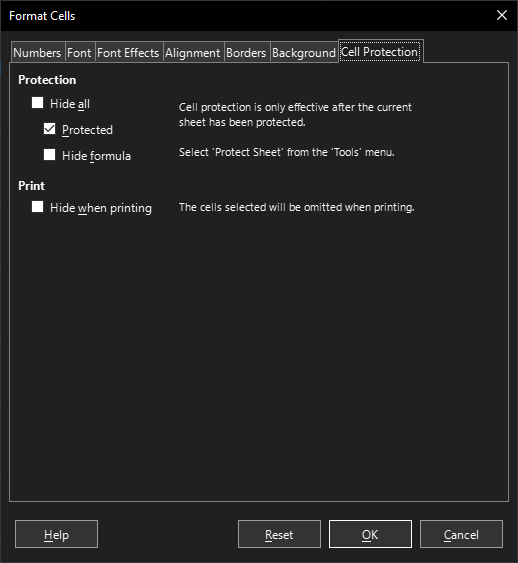
Fig. 342 Calc Format Cell dialog Cell Protection
Apply cell protection to a cell
Setup
from __future__ import annotations
import uno
from ooodev.calc import CalcDoc
from ooodev.loader import Lo
def main() -> int:
with Lo.Loader(connector=Lo.ConnectSocket()):
doc = CalcDoc.create_doc(visible=True)
sheet = doc.sheets[0]
Lo.delay(500)
doc.zoom_value(100)
cell = sheet["A1"]
cell.value = "Hello"
cell.style_protection(
hide_all=False,
hide_formula=True,
protected=True,
hide_print=True,
)
f_style = cell.style_protection_get()
assert f_style is not None
Lo.delay(1_000)
doc.close()
return 0
if __name__ == "__main__":
SystemExit(main())
Setting the cell protection
cell = sheet["A1"]
cell.value = "Hello"
cell.style_protection(
hide_all=False,
hide_formula=True,
protected=True,
hide_print=True,
)
Running the above code will produce the following output in Fig. 343.
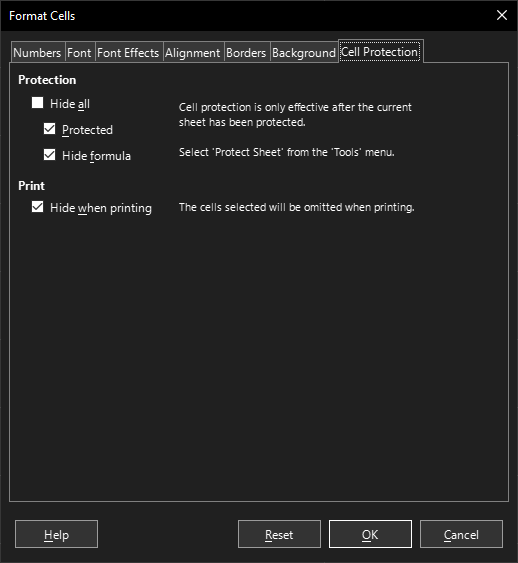
Fig. 343 Calc Format Cell dialog Cell Protection set
Getting cell protection from a cell
# ... other code
f_style = cell.style_protection_get()
assert f_style is not None
Apply cell protection to a range
Setup
from __future__ import annotations
import uno
from ooodev.calc import CalcDoc
from ooodev.loader import Lo
def main() -> int:
with Lo.Loader(connector=Lo.ConnectSocket()):
doc = CalcDoc.create_doc(visible=True)
sheet = doc.sheets[0]
Lo.delay(500)
doc.zoom_value(100)
rng = sheet.rng("A1:B1")
sheet.set_array(
values=[["Hello", "World"]], range_obj=rng
)
cell_rng = sheet.get_range(range_obj=rng)
cell_rng.style_protection(
hide_all=False,
hide_formula=True,
protected=True,
hide_print=True,
)
Lo.delay(1_000)
doc.close()
return 0
if __name__ == "__main__":
SystemExit(main())
Setting the range protection
# ... other code
cell_rng = sheet.get_range(range_obj=rng)
cell_rng.style_protection(
hide_all=False,
hide_formula=True,
protected=True,
hide_print=True,
)
Running the above code will produce the following output in Fig. 343.
Getting cell protection from a range
It is not recommended to get and instance of CellProtection
from a range.
This is because a range can have multiple cells with different cell protection settings and the CellProtection
may not properly represent the range.